Purpose:
The script is designed to provide a solution for closing all instances of major web browsers on a Windows machine using PowerShell.
It includes Microsoft Edge, Mozilla Firefox, Opera, and Google Chrome. The script enhances user interaction by including a fun countdown, offers customization through colour-coded messages, and improves error handling by catching and reporting issues during the process shutdown.
Script Breakdown:
Benefits:
Screenshot:
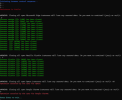
Kindly note that the N of No in the last line was accidentally cut off in the screenshot.
To ensure that the PowerShell script functions correctly, it must be run with administrative privileges.
This is because stopping processes on Windows often requires higher permissions than those granted to standard user accounts.
How to Run PowerShell as Administrator:
PowerShell scripts are governed by an execution policy that determines how scripts are run. To run the script, you might need to set the execution policy to allow script execution.
How to Check and Set Execution Policy:
Limiting Scope and Security Best Practices:
To enhance security while using PowerShell:
By following these guidelines, you can safely utilize PowerShell scripts to enhance productivity and perform administrative tasks efficiently while keeping your system secure.
This guide should help users understand the requirements and precautions necessary for safely running PowerShell scripts with administrative privileges.
The script is designed to provide a solution for closing all instances of major web browsers on a Windows machine using PowerShell.
It includes Microsoft Edge, Mozilla Firefox, Opera, and Google Chrome. The script enhances user interaction by including a fun countdown, offers customization through colour-coded messages, and improves error handling by catching and reporting issues during the process shutdown.
Script Breakdown:
- Introduction with Countdown:
- The script starts with a fun and engaging introduction that includes a countdown from 3 to 1. This part uses different text colours (blue for the countdown and dark red for the tagline "Resistance is futile!") to catch the user's attention and create a thematic lead-in to the task at hand.
- Function CheckAndCloseBrowser:
- This function is the core of the script. It takes two parameters: the friendly name of the browser ($browserName) and an array of process names associated with that browser ($processNames).
- It retrieves all running processes that match any name in the $processNames array. If any processes are found, it prompts the user with a warning that closing the browser will result in the loss of any unsaved data and asks for confirmation to proceed.
- If the user confirms (by typing "yes"), the script attempts to close each process forcefully. If a process cannot be closed (e.g., due to insufficient permissions), the script catches the exception and informs the user about the specific process that could not be terminated, including its ID and name.
- If no processes are found running for a browser, it informs the user accordingly.
- User Confirmation:
- The script seeks user confirmation before closing any browser to prevent accidental data loss. This feature ensures that users are aware of the consequences of terminating browser processes.
- Error Handling:
- The script includes try-catch blocks around the command that stop the browser processes. This helps manage situations where the script might otherwise fail due to issues like access denials. It ensures the script can handle such exceptions gracefully and inform the user about what went wrong.
- Final User Interaction:
- After handling all specified browsers, the script prompts the user to press Enter to exit. This allows users to review the output and ensures they do not miss any important information about the script's execution.
- Users need to run this script in PowerShell with administrative privileges to ensure it has the necessary permissions to manage and terminate processes.
- This script can be directly pasted into a PowerShell window or saved as a .ps1 file and executed by typing the path to the script file in PowerShell.
Benefits:
- This script is useful for any users who frequently need to close browser sessions cleanly and swiftly as part of troubleshooting, testing, or resetting browser environments. The user-friendly prompts and detailed feedback make it suitable even for less technical users who need a reliable way to manage browser processes.
Code:
# Introduction message with countdown
Write-Host "Initiating browser control sequence..." -ForegroundColor Blue
foreach ($i in 3..1) {
Write-Host "$i..."
Start-Sleep -Seconds 1
}
Write-Host "Resistance is futile!" -ForegroundColor DarkRed
Start-Sleep -Seconds 1
Write-Host "" # Adds an empty line for better visual separation
function CheckAndCloseBrowser($browserName, $processNames) {
Write-Host "" # Additional line for separation before each browser's processing starts
# Get all processes for the listed names
$processes = $processNames | ForEach-Object { Get-Process -Name $_ -ErrorAction SilentlyContinue } | Where-Object { $_ }
# Check if any processes are found
if ($processes) {
# Ask user for confirmation to close the browser
$confirmation = Read-Host "WARNING: Closing all open $browserName instances will lose any unsaved data. Do you want to continue? (yes/y or no/n)"
$confirmation = $confirmation.ToLower() # Convert input to lowercase
if ($confirmation -eq "yes" -or $confirmation -eq "y") {
foreach ($process in $processes) {
try {
$process | Stop-Process -Force -ErrorAction Stop
Write-Host "Process $($process.ProcessName) (ID: $($process.Id)) has been closed." -ForegroundColor Green
} catch {
Write-Host "Could not stop process $($process.ProcessName) (ID: $($process.Id)): Access is denied." -ForegroundColor Red
}
}
} elseif ($confirmation -eq "no" -or $confirmation -eq "n") {
Write-Host "Operation canceled by the user for $browserName." -ForegroundColor Red
} else {
Write-Host "Invalid input. Please enter 'yes/y' or 'no/n'." -ForegroundColor Yellow
return # Exit function if invalid input
}
} else {
Write-Host "No $browserName processes are running." -ForegroundColor Yellow
}
Write-Host "" # Additional line for separation after each browser's processing ends
}
# Check and close browsers
CheckAndCloseBrowser "Microsoft Edge" @("msedge", "MicrosoftEdgeUpdate", "msedgewebview2")
CheckAndCloseBrowser "Mozilla Firefox" @("firefox")
CheckAndCloseBrowser "Opera" @("opera")
CheckAndCloseBrowser "Google Chrome" @("chrome")
# Wait for user input to exit
Write-Host "Press Enter to exit..." -ForegroundColor Cyan
Read-Host
Screenshot:
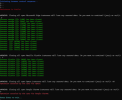
Kindly note that the N of No in the last line was accidentally cut off in the screenshot.
Important: Running the PowerShell Script as Administrator with Necessary Privileges
Running as Administrator:To ensure that the PowerShell script functions correctly, it must be run with administrative privileges.
This is because stopping processes on Windows often requires higher permissions than those granted to standard user accounts.
How to Run PowerShell as Administrator:
- Search for PowerShell in the Start menu.
- Right-click the PowerShell application.
- Select 'Run as administrator' from the context menu.
- If prompted by User Account Control (UAC), confirm that you want to allow the application to make changes to your device by clicking 'Yes'.
PowerShell scripts are governed by an execution policy that determines how scripts are run. To run the script, you might need to set the execution policy to allow script execution.
How to Check and Set Execution Policy:
- Open PowerShell as an administrator (as described above).
- To check the current execution policy, enter the following command:
Get-ExecutionPolicy
- If the policy is not set to RemoteSigned or Unrestricted (which are common policies for allowing script execution), set it by entering the following command:
Set-ExecutionPolicy RemoteSigned
- Confirm the action when prompted.
Limiting Scope and Security Best Practices:
To enhance security while using PowerShell:
- Avoid setting the execution policy to Unrestricted unless necessary.
RemoteSigned is typically sufficient and safer as it requires that scripts downloaded from the internet be signed by a trusted publisher.
- Consider using a restricted user account or a controlled environment for testing unknown scripts before running them on your main system.
- Maintain up-to-date antivirus software to protect against malware that might try to exploit scripts.
By following these guidelines, you can safely utilize PowerShell scripts to enhance productivity and perform administrative tasks efficiently while keeping your system secure.
This guide should help users understand the requirements and precautions necessary for safely running PowerShell scripts with administrative privileges.